This post was written 4-5 years ago, back in October 2014, but for some reason never left the draft folder. Well, here it is. Maybe this will give me the necessary incentive to produce a part 2 in the near future.
I’ve recently become interested in doing some small programming projects. Mainly using C and assembly language. For some reason low-level programming and embedded systems appeal to me.
For fun, I decided to poke into the code of Knights of the Sky, the PC-version. A game which has given me hours of fun when I was young. Being a 16-bit DOS based game I thought it would be a relative easy task. The actual source code isn’t publicly available to my knowledge, so for this I had to look from the outside and in using different tools like hex editor and disassembly.
How the game is assembled
The game is split into several executables, none of which I was able to start directly from Dosbox. Neither was I able to disassemble them into anything useful. A quick peek using a hex editor reveals that they are compressed with the LZ91 algorithm. This isn’t a problem, the internet is full of tools which can uncompress them and make executables which can be dissassembled.
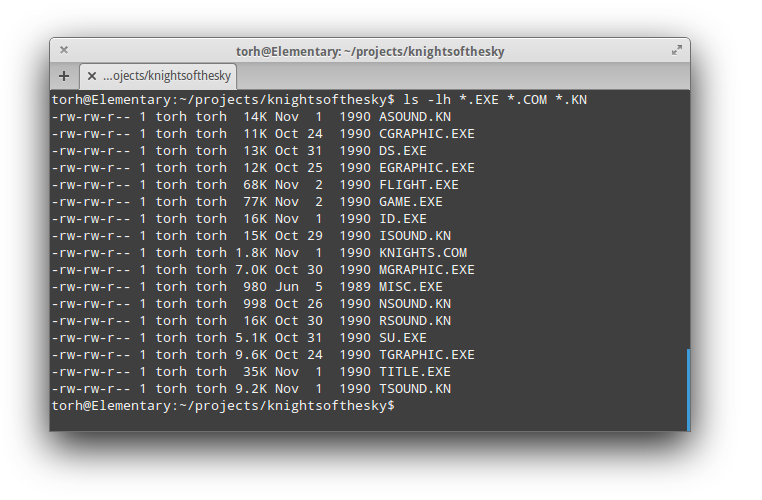
Splitting the files make some sense. Some because of the memory limit in early DOS era, but also because not all of the files are used. It all depends on the choices you make during setup, like sound and graphic options. Several of the executables have similar names, like CGRAPHIC.EXE, EGRAPHIC.EXE, MGRAPHIC.EXE and TGRAPHIC.EXE, and only one of them is used during game play. Same goes for sound.
Playing Knight of the Sky
To start the game the player would have to start KNIGHTS.COM, a basic binary executable which is loaded directly into memory location 0100h. This file acts as a loader which kicks off a chain of other executables.
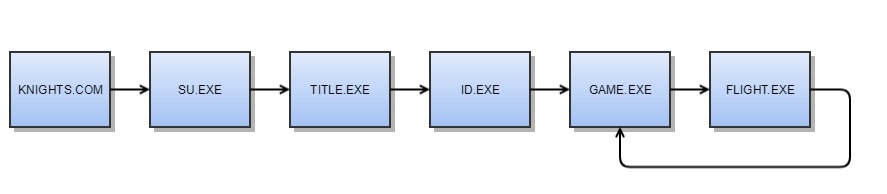
SU.EXE is the setup where the player can choose input (keyboard, mouse and/or joystick), sound and graphics options. This is passed along to DS.EXE which, and I’m guessing now, is the part responsible for the stack and the memory layout (remembering the choices made, etc). After SU.EXE, DS.EXE is run between every executable until the player exits the game.
Copy protection
ID.EXE is the game “copy protection” scheme, where you are presented with an image and have to choose the right option. This is found in the game manual. Remember those?
If the player selects wrong she or he is only able to play a training session. Again, I would guess that the result of this “copy protection” is also stored somewhere in memroy. MISC.EXE is also loaded into memory (overlay, not executed) by KNIGHTS.COM. This seems to be the code responsible for handling the game port (joustick). In my copy of the game this executable has a creation date of 1989, while the rest of the files are compiled in 1990.
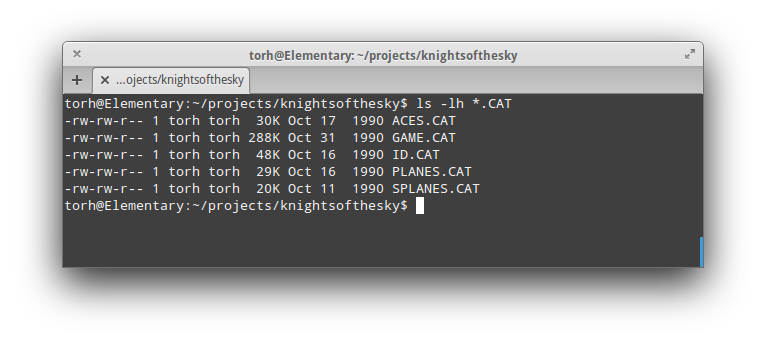
Going back to ID.EXE, there is also a file called ID.CAT, which is basically a container for the images used by this executable. It contains one background image and several logos. One of the logos are presented and the user has to select the correct name which belongs to that particular logo. Creating a program to extract those files was (almost) straight forward after using a hex editor to figure out the internal data structure.
The two first bytes tells how many files are “packed”, immediately followed by the following data structure times the number of the two first bytes. In this case, 18 times. The same structure is used for the other CAT-files in the game as well.
struct fileHeader {
char name[12]; // Filename with a maximum of 8.3, DOS-style
unsigned short u1; // ?? I Have no idea ??
unsigned int size; // File size in bytes
unsigned int offset; // Offset from beginning of file
}
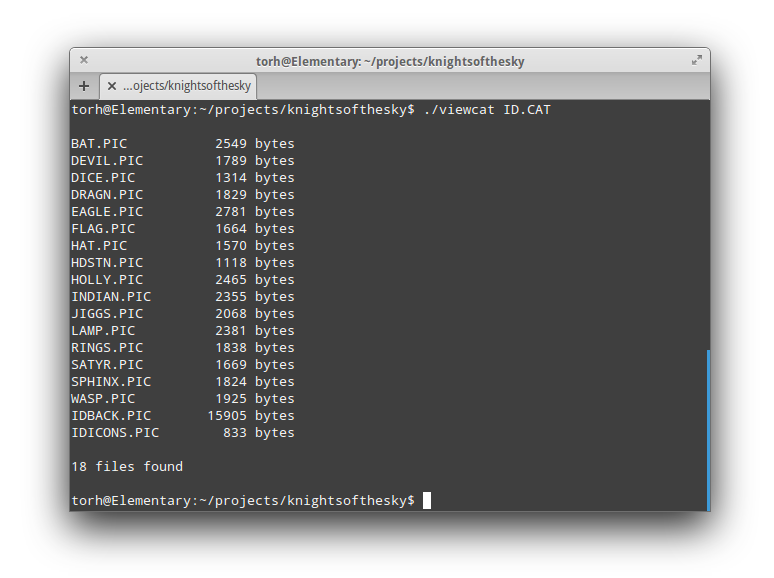
There are also some files ending with PLN, all of which has the same size (256 bytes) and almost the same content. The name is almost a dead give away that we’re talking about different colors, and swapping one file with another reveals that these are responsible for the color of your plane, seen from the outside (hitting F2 during flight) that is. However they do not seem to impact the landscape in any way. I’ll just assume that PLN is an abbreviation of the word “plane”.
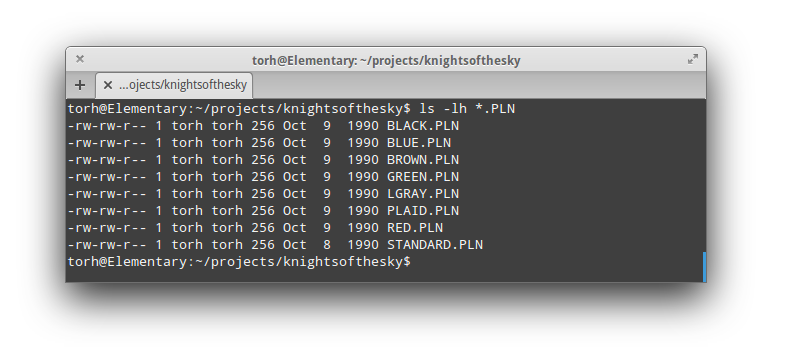
Savegame file
The last file I will mention is the ROSTER.DAT. This is the only file which changes during game play (did someone say save game?). It’s 35K bytes, and since the game supports 10 saved players (no more, no less), it was fairly easy to guess how much “space” each player occupies. The structure of this file has to be well-defined, but I have only scratched the surface so far. Then again, I’m more interested in decoding the graphics and sound then trying to cheat the game. It’s a pain to play without a joystick anyway.
Now that we know where the different files are used and why, we are ready to dive deeper and do some real disassembly. The focus next will be on extracting and viewing images as well as be able to play the music from the game.